#documentation
Rust
Rust samples
This article provides some code samples in Rust.
Command line interface
Command arguments
cargo run foo bar baz "This string is a sentence"
1 use std::env;
2
3 // cargo run hello world
4
5 fn main() {
6 let args: Vec<String> = env::args().collect();
7
8 // Retrieve first argument
9 let input = args[1].clone();
10
11 // Print all arguments
12 println!("{:?}", args); // ["target/debug/executable", "foo", "bar", "baz", "This string is a sentence"]
13 }
Standard input
1 use std::io;
2
3 fn main() {
4 // empty string
5 let mut input = String::new();
6
7 // Collecting user input
8 println!("Enter your name: ");
9 io::stdin()
10 .read_line(&mut input)
11 .expect("Failed to read line");
12
13 println!("Received name: {}", input);
14 }
15
Markdown
Sample article showcasing basic Markdown syntax and formatting for HTML elements.
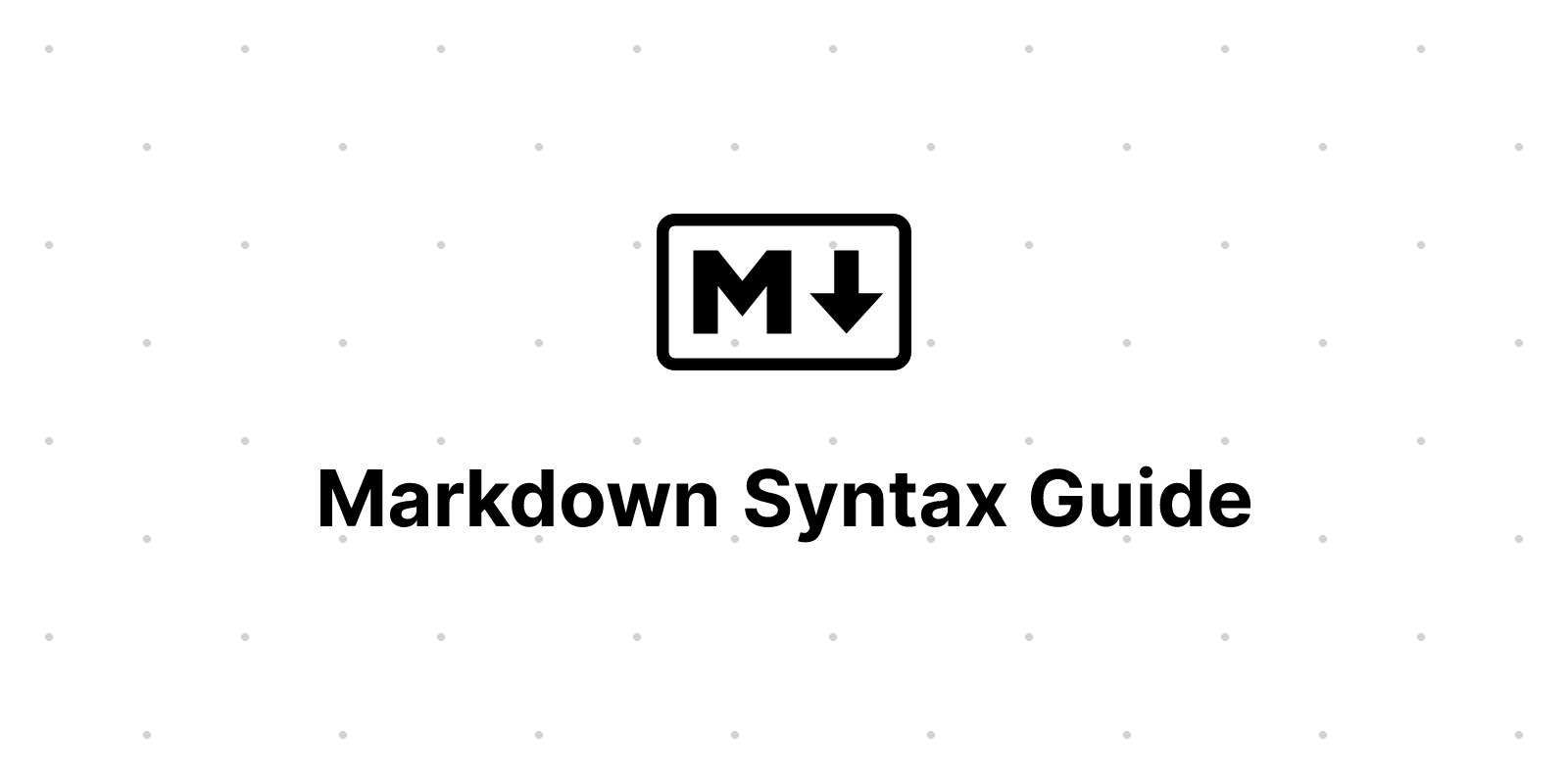
This article offers a sample of basic Markdown syntax that can be used in Zola content files, also it shows whether basic HTML elements are decorated with CSS in a Kita theme.
Headings
The following HTML <h1>
—<h6>
elements represent six levels of section headings. <h1>
is the highest section level while <h6>
is the lowest.
H1
H2
H3
H4
H5
H6
Paragraph
Xerum, quo qui aut unt expliquam qui dolut labo. Aque venitatiusda cum, voluptionse latur sitiae dolessi aut parist aut dollo enim qui voluptate ma dolestendit peritin re plis aut quas inctum laceat est volestemque commosa as cus endigna tectur, offic to cor sequas etum rerum idem sintibus eiur? Quianimin porecus evelectur, cum que nis nust voloribus ratem aut omnimi, sitatur? Quiatem. Nam, omnis sum am facea corem alique molestrunt et eos evelece arcillit ut aut eos eos nus, sin conecerem erum fuga. Ri oditatquam, ad quibus unda veliamenimin cusam et facea ipsamus es exerum sitate dolores editium rerore eost, temped molorro ratiae volorro te reribus dolorer sperchicium faceata tiustia prat.
Itatur? Quiatae cullecum rem ent aut odis in re eossequodi nonsequ idebis ne sapicia is sinveli squiatum, core et que aut hariosam ex eat.
Blockquotes
The blockquote element represents content that is quoted from another source, optionally with a citation which must be within a footer
or cite
element, and optionally with in-line changes such as annotations and abbreviations.
Blockquote without attribution
Tiam, ad mint andaepu dandae nostion secatur sequo quae. Note that you can use Markdown syntax within a blockquote.
Blockquote with attribution
Don't communicate by sharing memory, share memory by communicating.
— Rob Pike1
The above quote is excerpted from Rob Pike's talk during Gopherfest, November 18, 2015.
Links
To create a link, enclose the link text in brackets and then follow it immediately with the URL in parentheses.
To quickly turn a URL or email address into a link, enclose it in angle brackets.
Images
Tables
Tables aren't part of the core Markdown spec, but Zola supports supports them out-of-the-box.
Name | Age |
---|---|
Bob | 27 |
Alice | 23 |
Inline Markdown within tables
Italics | Bold | Code |
---|---|---|
italics | bold | code |
Code Blocks
Code block with backticks
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8" />
<title>Example HTML5 Document</title>
</head>
<body>
<p>Test</p>
</body>
</html>
Code block indented with four spaces
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Example HTML5 Document</title>
</head>
<body>
<p>Test</p>
</body>
</html>
List Types
Ordered List
- First item
- Second item
- Third item
Unordered List
- List item
- Another item
- And another item
Nested list
- Fruit
- Apple
- Orange
- Banana
- Dairy
- Milk
- Cheese
Other Elements — abbr, sub, sup, kbd, mark
GIF is a bitmap image format.
H2O
Xn + Yn = Zn
Press CTRL+ALT+Delete to end the session.
Most salamanders are nocturnal, and hunt for insects, worms, and other small creatures.
Zola Syntax Highlighting
Syntax Highlighting in Zola.
Zola comes with built-in syntax highlighting but you first need to enable it in the configuration.
Annotations
You can use additional annotations to customize how code blocks are displayed:
linenos
to enable line numbering.
```rust,linenos
use highlighter::highlight;
use std::env;
let code = "...";
highlight(code);
fn foo(bar: i32) {
println!("{}", bar);
let baz = bar + 42;
println!("{}", baz);
println!("{}", baz * 3.14);
}
```
1 use highlighter::highlight;
2 use std::env;
3 let code = "...";
4 highlight(code);
5 fn foo(bar: i32) {
6 println!("{}", bar);
7 let baz = bar + 42;
8 println!("{}", baz);
9 println!("{}", baz * 3.14);
10 }
linenostart
to specify the number for the first line (defaults to 1)
```rust,linenos,linenostart=20
use highlighter::highlight;
use std::env;
let code = "...";
highlight(code);
fn foo(bar: i32) {
println!("{}", bar);
let baz = bar + 42;
println!("{}", baz);
println!("{}", baz * 3.14);
}
```
20 use highlighter::highlight;
21 use std::env;
22 let code = "...";
23 highlight(code);
24 fn foo(bar: i32) {
25 println!("{}", bar);
26 let baz = bar + 42;
27 println!("{}", baz);
28 println!("{}", baz * 3.14);
29 }
hl_lines
to highlight lines. You must specify a list of inclusive ranges of lines to highlight, separated bylinenostart
doesn't influence the values, it always refers to the codeblock line number.
```rust,hl_lines=1 3-5 9
use highlighter::highlight;
use std::env;
let code = "...";
highlight(code);
fn foo(bar: i32) {
println!("{}", bar);
let baz = bar + 42;
println!("{}", baz);
println!("{}", baz * 3.14);
}
```
use highlighter::highlight;
use std::env;
let code = "...";
highlight(code);
fn foo(bar: i32) {
println!("{}", bar);
let baz = bar + 42;
println!("{}", baz);
println!("{}", baz * 3.14);
}
hide_lines
to hide lines. You must specify a list of inclusive ranges of lines to hide, separated by
```rust,hide_lines=1-2
use highlighter::highlight;
use std::env;
let code = "...";
highlight(code);
fn foo(bar: i32) {
println!("{}", bar);
let baz = bar + 42;
println!("{}", baz);
println!("{}", baz * 3.14);
}
```
let code = "...";
highlight(code);
fn foo(bar: i32) {
println!("{}", bar);
let baz = bar + 42;
println!("{}", baz);
println!("{}", baz * 3.14);
}
Kita Shortcodes
The Kita theme shortcodes.
The Kita theme providers multiple shortcodes.
Never heard of shortcodes? See Zola documentation for more information.
Mermaid
To use Mermaid in your page, you have to set extra.mermaid = true
in the frontmatter of page.
+++
title = "Your page title"
[extra]
mermaid = true
+++
Then you can use the mermaid()
shortcodes like:
</span><span>
</span><span>graph TD;
</span><span>A-->B;
</span><span>A-->C;
</span><span>B-->D;
</span><span>C-->D;
</span><span>
</span><span>
This will be rendered as:
graph TD; A-->B; A-->C; B-->D; C-->D;
In addition, you can use code block inside mermaid()
shortcodes and the code block will be ignored.
The code block prevents formatter from breaking mermaid's formatting.
</span><span>
</span><span>```</span><span style="color:#bd93f9;">mermaid
</span><span>sequenceDiagram
</span><span> participant Alice
</span><span> participant Bob
</span><span> Alice->>John: Hello John, how are you?
</span><span> loop Healthcheck
</span><span> John->>John: Fight against hypochondria
</span><span> end
</span><span> Note right of John: Rational thoughts <br/>prevail!
</span><span> John-->>Alice: Great!
</span><span> John->>Bob: How about you?
</span><span> Bob-->>John: Jolly good!
</span><span>```
</span><span>
</span><span>
This will be rendered as:
sequenceDiagram participant Alice participant Bob Alice->>John: Hello John, how are you? loop Healthcheck John->>John: Fight against hypochondria end Note right of John: Rational thoughts <br/>prevail! John-->>Alice: Great! John->>Bob: How about you? Bob-->>John: Jolly good!
Admonition
The admonition()
shortcode displays a banner to help you put notice in your page.
You can use the admonition()
shortcode like:
{%/ admonition(type="tip", title="tip") *%}
The `tip` admonition.
{%* end *%}
The admonition shortcode has 12 different types:
The note
admonition.
The abstract
admonition.
The info
admonition.
The tip
admonition.
The success
admonition.
The question
admonition.
The warning
admonition.
The failure
admonition.
The danger
admonition.
The bug
admonition.
The example
admonition.
The quote
admonition.
Gallery
The gallery()
shortcode is very simple html-only clickable picture gallery that displays all images from the page assets.
It's from Zola documentation
{{# gallery() #}}
Samba Installation
Configuring Samba on a new system
Instructions from the Ubuntu Server docs 1.
Installation
sudo apt install samba
Configuration on Ubuntu
Add the following files at the end of /etc/samba/smb.conf
.
[<share-name>]
path = </path/to/folder>
valid users = <username>
read only = no
For example,
[server1-project]
path = /path/to/project
valid users = ubuntu
read only = no
Enable the configuration
Restart the Samba services
sudo systemctl restart smbd.service nmbd.service
Configuration on Fedora
On Fedora, here is a tutorial 2 and here are the docs 3.